Ultimate Attributes Pack
Ultimate Attributes Pack is a unity package of 37 new usefull attributes to improve your project inspectors. It expand the possibilities you have in inspectors by displaying variables with many unique properties, or also some decorators like titles, subtitles or lines to improve the understanding of your scripts.
All the attributes are included in the UltimateAttributesPack namespace.
- Decorators
- Variable aspect
- Buttons
- Folded infos
- Conditionals
- Validators
- Components getters
- Popups
- Online documentation
- PDF documentation
- Example scene included with an example script for each attribute
The attributes and their parameters are made with the goal to be very easy and quick to use, but with many possible customization. Many attributes have predefined parameters so don't hesitate to check them to fully custom them as you want.
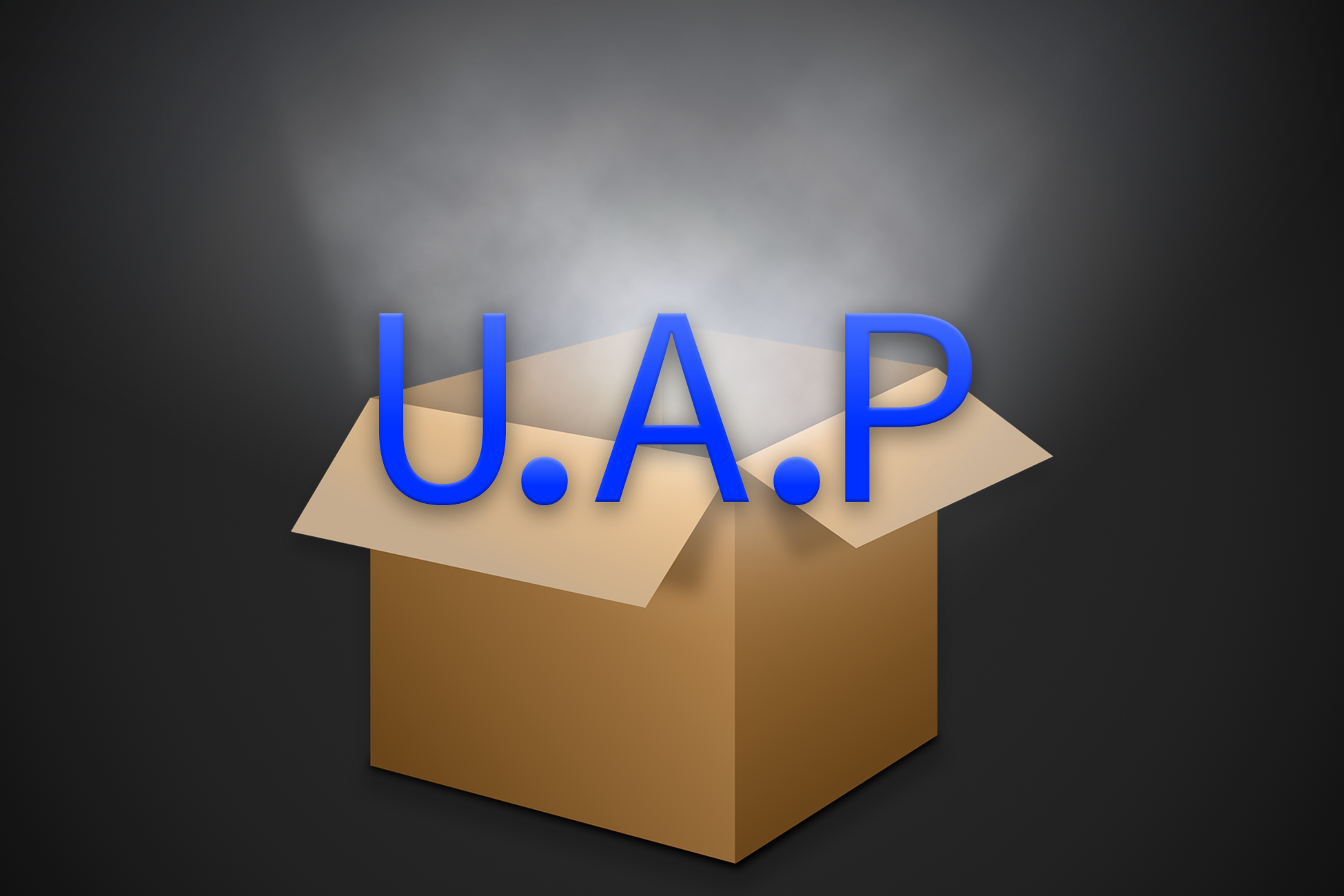
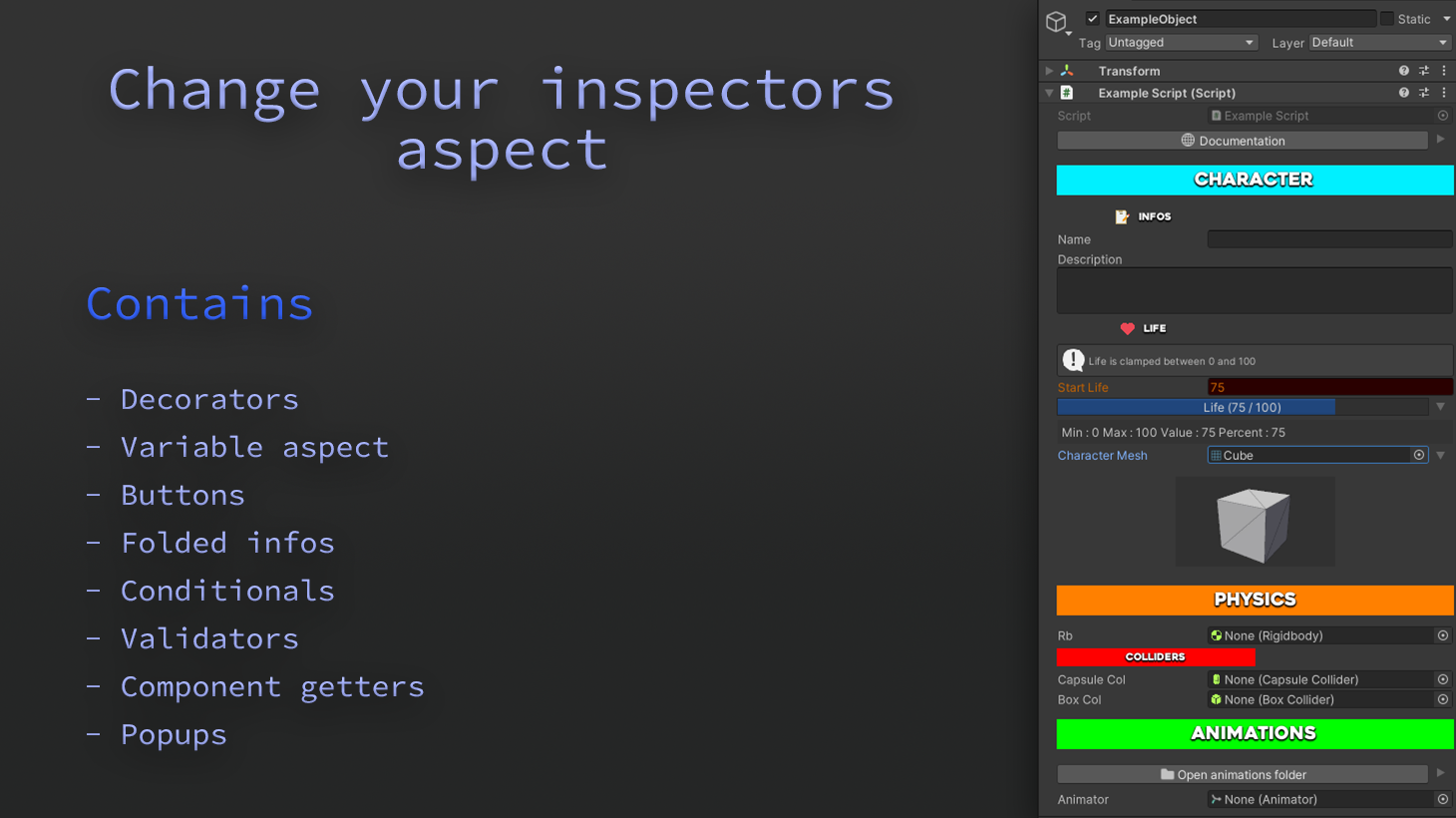
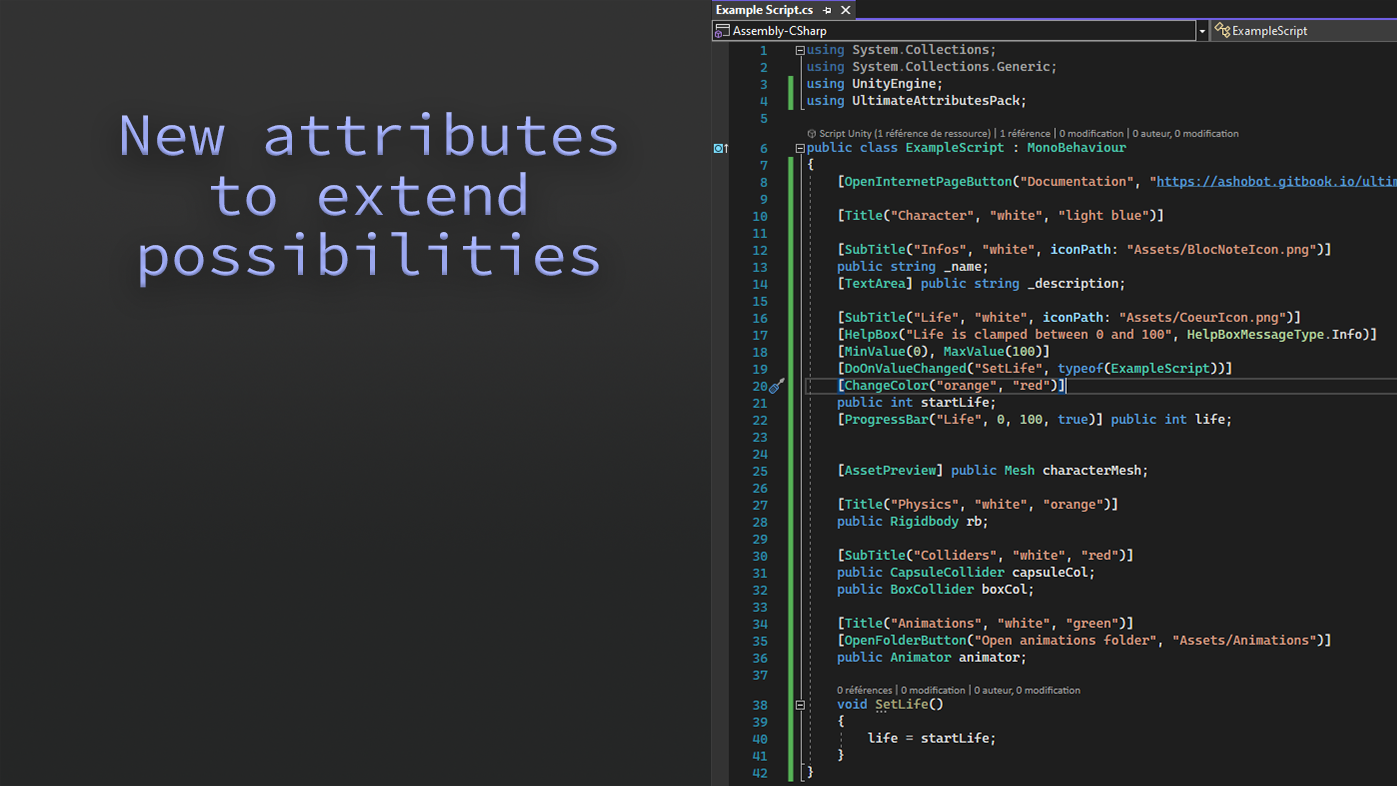
13,80$
Buy on Unity Asset StoreDocumentation
Title
Displays a big title in the inspector to create different sections.
Everything.
- Title
- TextColor
- BackgroundColor
- IconPath
using UltimateAttributesPack;
public class TitleExample : MonoBehaviour
{
// If color is not defined, it use the base text color of the inspector
[Title("Float")]
public float firstFloat;
public float secondFloat;
// You can use predefined colors ("black", "blue", "cyan", "green", "grey", "magenta", "red", "white", "yellow", "orange", "pink", "purple", "light green", "light blue", "brown", "dark blue")
[Title("Integers", "white", "light blue")]
public int firstInt;
public int secondInt;
// You can also use exadecimal for the color
[Title("Booleans", "E6B997", "FFFBDC")]
public bool firstBool;
public bool secondBool;
// You can set an icon path that will be displayed at the left of the title
[Title("Strings", "444746", "grey", "Assets/UltimateAttributesPack/Examples/Assets/Icons/PenIcon.png")]
public string firstString;
public string secondString;
}
SubTitle
Displays a subtitle in the inspector to create different sub-sections.
Everything.
- Title
- TextColor
- BackgroundColor
- IconPath
using UltimateAttributesPack;
public class SubTitleExample : MonoBehaviour
{
// If color is not defined, it use the base text color of the inspector
[SubTitle("Float")]
public float firstFloat;
public float secondFloat;
// You can use predefined colors ("black", "blue", "cyan", "green", "grey", "magenta", "red", "white", "yellow", "orange", "pink", "purple", "light green", "light blue", "brown", "dark blue")
[SubTitle("Integers", "white", "light blue")]
public int firstInt;
public int secondInt;
// You can also use exadecimal for the color
[SubTitle("Booleans", "E6B997", "FFFBDC")]
public bool firstBool;
public bool secondBool;
// You can set a icon path that will be displayed at the left of the subtitle
[SubTitle("Strings", "444746", "grey", "Assets/UltimateAttributesPack/Examples/Assets/Icons/PenIcon.png")]
public string firstString;
public string secondString;
}
LineTitle
Displays a line title in the inspector to create different sections.
Everything.
- Title
- TextColor
- LineColor
using UltimateAttributesPack;
public class LineTitleExample : MonoBehaviour
{
// If color is not defined, it use the base text color of the inspector
[LineTitle("Float")]
public float firstFloat;
public float secondFloat;
// You can use predefined colors ("black", "blue", "cyan", "green", "grey", "magenta", "red", "white", "yellow", "orange", "pink", "purple", "light green", "light blue", "brown", "dark blue")
[LineTitle("Integers", "white", "light blue")]
public int firstInt;
public int secondInt;
// You can also use exadecimal for the color
[LineTitle("Booleans", "E6B997", "FFFBDC")]
public bool firstBool;
public bool secondBool;
}
Line
Displays a line in the inspector to separate sections.
Everything.
- Color
using UltimateAttributesPack;
public class LineExample : MonoBehaviour
{
// If color is not defined, it use the base text color of the inspector
[Line]
public int intValue;
// You can use predefined colors ("black", "blue", "cyan", "green", "grey", "magenta", "red", "white", "yellow", "orange", "pink", "purple", "light green", "light blue", "brown", "dark blue")
[Line("light blue")]
public float floatValue;
// You can also use exadecimal for the color
[Line("34A853")]
public Vector3 vector;
}
HelpBox
Displays an helpbox in the inspector to show additional informations.
Everything.
- HelpText
- MessageType
using UltimateAttributesPack;
public class HelpBoxExample : MonoBehaviour
{
// You can show an helpbox without message type to just show the text
[HelpBox("This is an helpbox", HelpBoxMessageType.None)]
public bool boolValue;
// If you don't define message type, it display an info helpbox
[HelpBox("This is an info helpbox")]
public int intValue;
// You can set the message type as you want (None, Info, Warning, Error)
[HelpBox("This is a warning helpbox", HelpBoxMessageType.Warning)]
public float floatValue;
[HelpBox("This is an error helpbox", HelpBoxMessageType.Error)]
public string stringValue;
}
SetIcon
Displays an icon at the left of the variable.
Everything.
- IconPath
- IconSize
using UltimateAttributesPack;
public class SetIconExample : MonoBehaviour
{
// SetIcon attribute displays an icon at the left of the variable
[SetIcon("Assets/UltimateAttributesPack/Examples/Assets/Icons/PenIcon.png")]
public string stringValue;
// You can also change the icon size (small, medium or large)
[SetIcon("Assets/UltimateAttributesPack/Examples/Assets/Icons/PenIcon.png", IconSize.Large)]
public string secondStringValue;
}
ChangeColor
Change the color of the variable (text or/and background).
Everything.
- TextColor
- BackgroundColor
using UltimateAttributesPack;
public class ChangeColorExample : MonoBehaviour
{
// You can change the text color and the background color of the variable
[ChangeColor("red", "orange")]
public string stringValue;
// It also works hexadecimal
[ChangeColor("C2E7FF", "444746")]
public float floatValue;
}
ChangeLabel
Change the label of the variable.
Everything.
- NewLabel
using UltimateAttributesPack;
public class ChangeLabelExample : MonoBehaviour
{
// ChangeLabel attribute change the label of the variable in the inspector.
[ChangeLabel("New Label")]
public string stringValue; // The variable name is "stringValue" but the label displayed in the inspector is "New Label"
}
Indent
Indent the variable to a target level in the inspector.
Everything.
- IndentLevel
using UltimateAttributesPack;
public class IndentExample : MonoBehaviour
{
public string stringValue;
// Indent attribute can be used to indent the variable to a specific level in the inspector
[Indent(2)]
public float floatValue;
[Indent(4)]
public float intValue;
}
Prefix
Displays a prefix text at the left of the variable in the inspector.
Everything.
- PrefixText
using UltimateAttributesPack;
public class PrefixExample : MonoBehaviour
{
// Prefix attribute displays a prefix text at the left of the variable
[Prefix("Prefix")]
public string stringValue;
}
Suffix
Displays a suffix text at the right of the variable in the inspector.
Everything.
- SuffixText
using UltimateAttributesPack;
public class SuffixExample : MonoBehaviour
{
// Suffix attribute displays a suffix text at the right of the variable
[Suffix("Suffix")]
public string stringValue;
}
ReadOnly
Displays the variable in read only in the inspector.
Everything.
- None.
using UltimateAttributesPack;
public class ReadOnlyExample : MonoBehaviour
{
// It shows the variable in readonly in the inspector
[ReadOnly]
public float floatValue;
}
MinMaxSlider
Displays a MinMaxSlider for Vector2 or Vector2Int in the inspector.
- Vector2
- Vector2Int
- Min
- Max
using UltimateAttributesPack;
public class MinMaxSliderExample : MonoBehaviour
{
// MinMaxSliders are principally used to randomize between a min and a max values
// You can use it on vector2
[MinMaxSlider(0, 100)]
public Vector2 vector2;
// You can also use it on vector2Int
[MinMaxSlider(0, 100)]
public Vector2Int vector2Int;
}
ProgressBar
Displays a progress bar in the inspector with min and max values, and based on variable value.
- Float
- Int
- Text
- Min
- Max
- DisplayPercent
using UltimateAttributesPack;
public class ProgressBarExample : MonoBehaviour
{
// To change the progress bar value, you can use the FunctionButton attribute
[FunctionButton("Reset progress bar value", "ResetProgressBar", typeof(ProgressBarExample))]
// You can also use the DoOnValueChanged attribute
[DoOnValueChanged("SetProgressBar", typeof(ProgressBarExample))]
public float currentValue;
// You can use it to display a bar on the inspector to visualize the value between a min and a max
[ProgressBar("Float value", 0, 100)]
public float floatProgressBar;
// You can also display the current percent of the value on the title
[ProgressBar("Int value", 0, 100, true)]
public int intProgressBar;
void SetProgressBar()
{
floatProgressBar = currentValue;
intProgressBar = Mathf.RoundToInt(currentValue);
}
void ResetProgressBar()
{
currentValue = 0;
floatProgressBar = 0;
intProgressBar = 0;
}
}
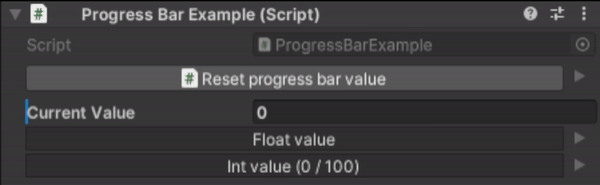
FunctionButton
Displays a button in the inspector that execute a function without parameters when clicked.
Everything.
- Text
- FunctionName
- ClassType
using UltimateAttributesPack;
public class FunctionButtonExample : MonoBehaviour
{
// You can use it to execute a function of the script in editor or play mode
[FunctionButton("Click to log a message in console", "LogMessage", typeof(FunctionButtonExample))]
public float floatValue;
[FunctionButton("Click to increment value", "IncrementValue", typeof(FunctionButtonExample))]
public int intValue;
// You can also use it to call a function on another script of the object
[FunctionButton("Click to log a message from another script of the object", "LogMessageOfOtherScript", typeof(DoOnValueChangedExample))]
public string stringValue;
void LogMessage()
{
Debug.Log("This is a message in the console");
}
void IncrementValue()
{
intValue++;
}
}
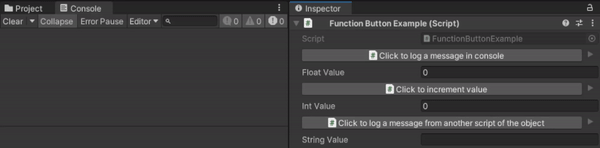
OpenFolderButton
Displays a button in the inspector that open a specific project folder when clicked.
Everything.
- Text
- FolderPath
using UltimateAttributesPack;
public class OpenFolderButtonExample : MonoBehaviour
{
// You can use it to open a specific folder of the project
[OpenFolderButton("Click to open the UltimateAttributesPack root folder", "Assets/UltimateAttributesPack")]
public float floatValue;
}
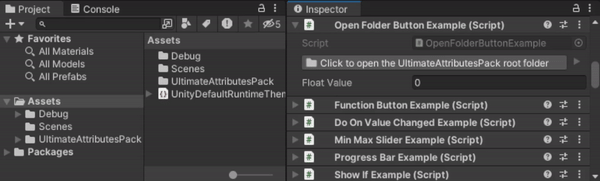
OpenInternetPageButton
Displays a button in the inspector that open a specific internet page when clicked.
Everything.
- Text
- Link
using UltimateAttributesPack;
public class OpenInternetPageButtonExample : MonoBehaviour
{
// You can use it in different ways, like redirecting to an online documentation or a specific video
[OpenInternetPageButton("Click to open a specific internet page", "https://assetstore.unity.com/")]
public float floatValue;
}
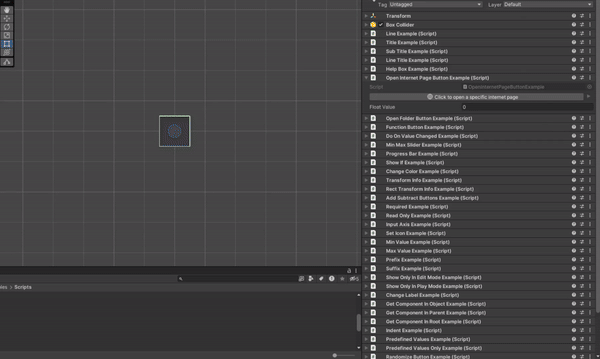
RandomizeButton
Displays a button at the right of the variable in the inspector that randomize it between a minimum, a maximum and a digits count when clicked.
- Float
- Int
- MinValue
- MaxValue
- Digits
using UltimateAttributesPack;
public class RandomizeButtonExample : MonoBehaviour
{
// RandomizeButton attribute displays a button at the right of the variable to randomize the value between a min and a max and with a digits count
// Digits count have a maximum of 5
[RandomizeButton(0, 100, 3)]
public float floatValue;
// It also works with int
[RandomizeButton(-50, 50, 0)]
public int intValue;
}

AddSubtractButtons
Displays two buttons in the inspector that add and subtract defined values to the variable when clicked.
- Float
- Int
- SubtractValue
- AddValue
using UltimateAttributesPack;
public class AddSubtractButtonsExample : MonoBehaviour
{
// AddSubtractButtons attribute displays buttons at the right of the variable to add of subtract it with specific values
[AddSubtractButtons(1, 1)]
public int intValue;
// It also works with float values
[AddSubtractButtons(2.5f, 2.5f)]
public float floatValue;
}

AssetPreview
Displays a folded interractive preview of the game object, the mesh or the image (Sprite, Texture, Texture2D) in the inspector.
- GameObject
- Mesh
- Sprite
- Texture
- Texture2D
- PreviewSize
using UltimateAttributesPack;
public class AssetPreviewExample : MonoBehaviour
{
// AssetPreview attribute displays a interactive preview of a mesh, a gameobject or an image (Sprite, Texture, Texture2D)
[AssetPreview]
public Mesh specificMesh;
// You can also change the size of the preview
[AssetPreview(PreviewSize.Large)]
public GameObject specificObject;
[AssetPreview(PreviewSize.Medium)]
public Texture specificTexture;
}
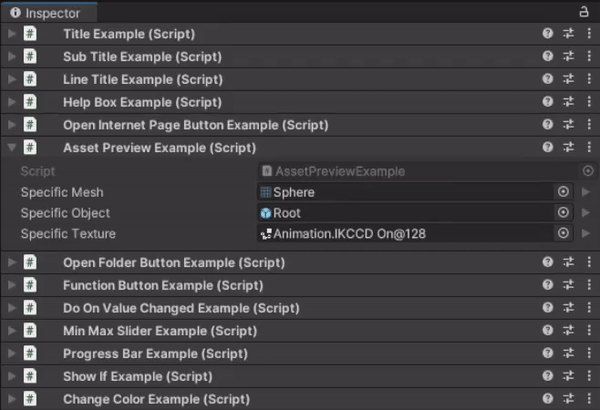
TransformInfo
Displays a folded menu with all the values of the Transform in the inspector.
- Transform
- CanModifyValues
using UltimateAttributesPack;
public class TransformInfoExample : MonoBehaviour
{
// If you use TransformInfo attribute, it displays a folfout menu with all the values of the transform
[TransformInfo]
public Transform transformValue;
// You can set the param to true to modify the Transform values in the foldout menu
[TransformInfo(true)]
public Transform transformValueModifiable;
}
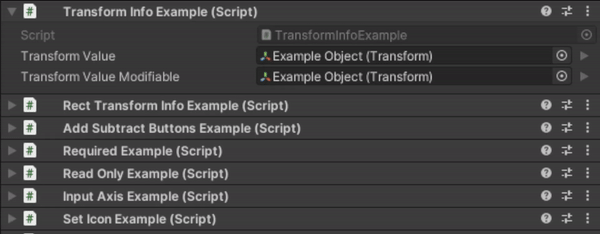
RectTransformInfo
Displays a folded menu with all the values of the RectTransform in the inspector.
- RectTransform
- CanModifyValues
using UltimateAttributesPack;
public class RectTransformInfoExample : MonoBehaviour
{
// If you use RectTransformInfo attribute, it displays a folfout menu with all the values of the RectTransform
[RectTransformInfo]
public RectTransform rectTransformValue;
// You can set the param to true to modify the RectTransform values in the foldout menu
[RectTransformInfo(true)]
public RectTransform rectTransformValueModifiable;
}
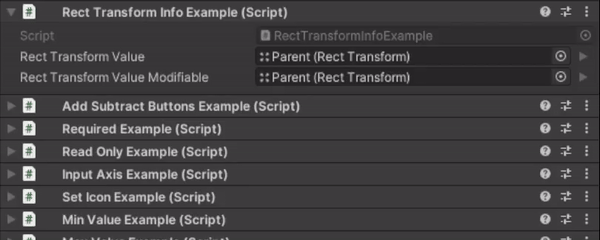
ShowIf
Displays the variable in the inspector only if the condition set with ComparisonType parameter is true between compared variable and condition value.
Everything.
- ComparedVariableName
- ConditionValue
- ComparisonType
- DisablingType
Compared variable and condition value must be the same type and only work with bool, enum, float, int and string.
using UltimateAttributesPack;
public class ShowIfExample : MonoBehaviour
{
public bool showFloat;
[ShowIf("showFloat", true)] // You can use it to show variables if a bool is on a specific state
public float floatValue;
[Space]
public float showIntIfSuperiorTo5;
[ShowIf("showIntIfSuperiorTo5", 5, ComparisonType.GreaterThan)] // You can also use it with numeric comparison
public int intValue;
[Space]
public string showStringIfEqualTo4;
[ShowIf("showStringIfEqualTo4", 4, ComparisonType.Equals)] // It also works with string lenght (characters number is the value)
public string stringValue;
[Space]
public string writeYesToShow = "Yes";
[ShowIf("writeYesToShow", "Yes", ComparisonType.Equals)] // It also works with string comparison
public string secondStringValue;
[Space]
public bool readOnlyShowIf;
[ShowIf("readOnlyShowIf", true, ComparisonType.Equals, DisablingType.ReadOnly)] // You can also set the disabling type to readonly if you want it to be visible in readonly in the inspector when it's disabled
public string thirdStringValue;
}
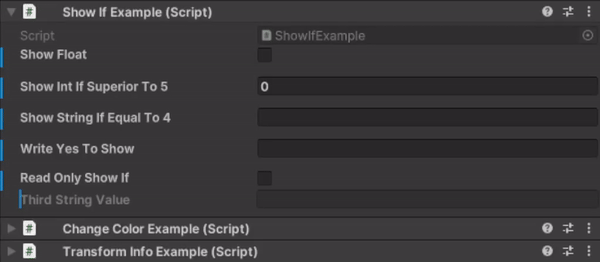
ShowOnlyInEditMode
Displays the variable in the inspector only if the project is in edit mode.
Everything.
- DisablingType
using UltimateAttributesPack;
public class ShowOnlyInEditModeExample : MonoBehaviour
{
// ShowOnlyInEditMode displays the variable only in edit mode
[ShowOnlyInEditMode]
public float floatValue;
// You can also set the param to ReadOnly to show the variable in readonly when it's disabled
[ShowOnlyInEditMode(DisablingType.ReadOnly)]
public int intValue;
}
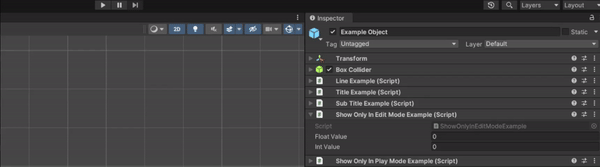
ShowOnlyInPlayMode
Displays the variable in the inspector only if the project is in play mode.
Everything.
- DisablingType
using UltimateAttributesPack;
public class ShowOnlyInPlayModeExample : MonoBehaviour
{
// ShowOnlyInEditMode displays the variable only in edit mode
[ShowOnlyInPlayMode]
public float floatValue;
// You can also set the param to ReadOnly to show the variable in readonly when it's disabled
[ShowOnlyInPlayMode(DisablingType.ReadOnly)]
public int intValue;
}
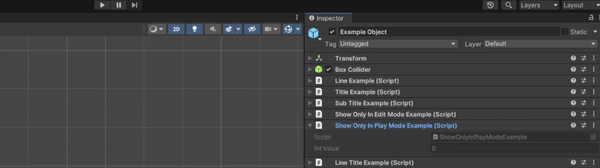
DoOnValueChanged
Executes a function if the variable change.
Everything.
- FunctionName
- ClassType
using UltimateAttributesPack;
public class DoOnValueChangedExample : MonoBehaviour
{
// You can use it to execute a function if a value has changed
[DoOnValueChanged("LogMessageOnValueChanged", typeof(DoOnValueChangedExample))]
public float floatValue;
void LogMessageOnValueChanged()
{
Debug.Log("The value has changed");
}
}
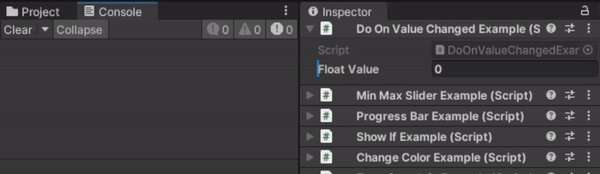
Required
Displays an error helpbox above the variable if it's empty.
- String
- Object Reference
- Exposed Reference
- Managed Reference
- LogError
using UltimateAttributesPack;
public class RequiredExample : MonoBehaviour
{
// Required attribute displays an helpbox in inspector if the variable is null
[Required]
public GameObject exampleObject;
// You can also set the param to true to log an error in the console if the variable is null
[Required(true)]
public GameObject secondExampleObject;
}
MinValue
Clamps the variable to a minimum value.
- Float
- Int
- MinValue
using UltimateAttributesPack;
public class MinValueExample : MonoBehaviour
{
// MinValue attribute clamp the variable to a minimum
[MinValue(0)]
public int intValue;
// It also works with floats
[MinValue(2.5f)]
public float floatValue = 2.5f;
}
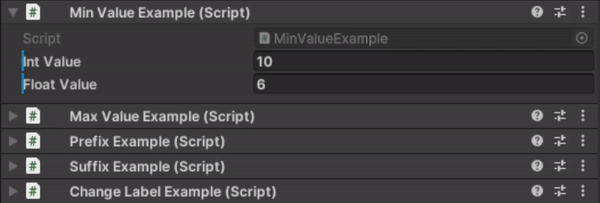
MaxValue
Clamps the variable to a maximum value.
- Float
- Int
- MaxValue
using UltimateAttributesPack;
public class MaxValueExample : MonoBehaviour
{
// MaxValue attribute clamp the variable to a maximum
[MaxValue(5)]
public int intValue;
// It also works with floats
[MaxValue(2.5f)]
public float floatValue;
}
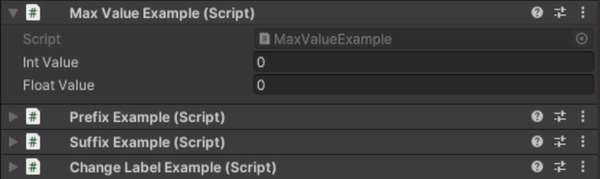
GetComponentInObject
Takes automatically the target component in the object.
- Object Reference
- Exposed Reference
- Managed Reference
- CreateOneIfEmpty
Can't be used on arrays and lists.
using UltimateAttributesPack;
public class GetComponentInObjectExample : MonoBehaviour
{
// GetComponentInObject attribute can be used to automatically get the target component of the object and set the variable with it
[GetComponentInObject]
public BoxCollider boxColliderOfObject;
// You can set the param to true to create a new component in the object automatically if there is none
// --> [GetComponentInObject(true)]
}
GetComponentInParent
Takes automatically the target component in the object's parent.
- Object Reference
- Exposed Reference
- Managed Reference
- CreateOneIfEmpty
Can't be used on arrays and lists.
using UltimateAttributesPack;
public class GetComponentInParentExample : MonoBehaviour
{
// GetComponentInParent attribute can be used to automatically get the target component of the object's parent and set the variable with it
[GetComponentInParent]
public BoxCollider boxColliderOfParent;
// You can set the param to true to create a new component in the object's parent automatically if there is none
// --> [GetComponentInParent(true)]
}
GetComponentInRoot
Takes automatically the target component in the object's root.
- Object Reference
- Exposed Reference
- Managed Reference
- CreateOneIfEmpty
Can't be used on arrays and lists.
using UltimateAttributesPack;
public class GetComponentInRootExample : MonoBehaviour
{
// GetComponentInRoot attribute can be used to automatically get the target component of the object's root and set the variable with it
[GetComponentInRoot]
public BoxCollider boxColliderOfRoot;
// You can set the param to true to create a new component in the object's root automatically if there is none
// --> [GetComponentInRoot(true)]
}
InputAxis
Displays a popup in the inspector to select an input axis.
- String
- Int
None.
using UltimateAttributesPack;
public class InputAxisExample : MonoBehaviour
{
// Shows a popup menu to select a specific input axis (returns the index of the input axis)
[InputAxis]
public int intInputAxis;
// It can also be used on string variables to return the name of the input axis
[InputAxis]
public string stringInputAxis;
}
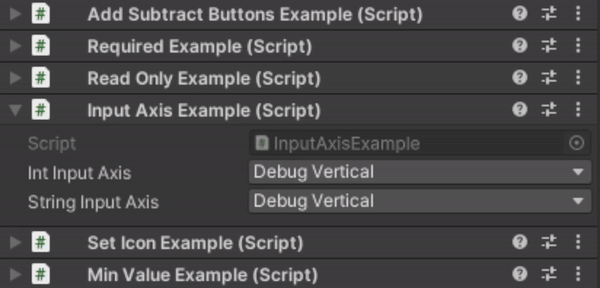
Tag
Displays a popup in the inspector to select a tag.
- String
None.
using UltimateAttributesPack;
public class TagExample : MonoBehaviour
{
// Tag attribute displays a popup in the inspector to select a tag (only works with string)
[Tag]
public string tagName; // Returns the tag name
}
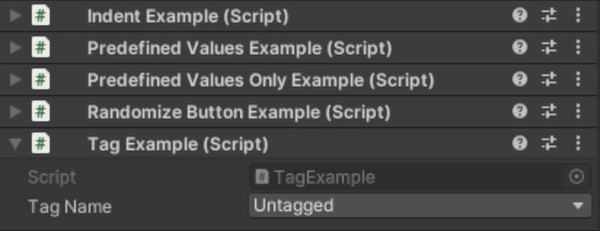
Scene
Displays a popup in the inspector to select a scene.
- String
- Int
None.
using UltimateAttributesPack;
public class SceneExample : MonoBehaviour
{
// Scene attribute dislays a popup in the inspector to select a scene (scenes must be in build settings)
[Scene]
public int sceneIndex; // Returns the index of the scene if the variable is an int
[Scene]
public string sceneName; // Returns the name of the scene if the variable is a string
}
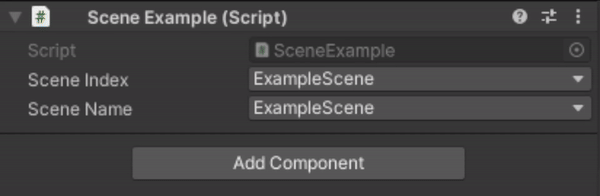
PredefinedValues
Displays a button at the right of the variable in the inspector to select a predefined value in parameters. The variable can be changed to a value that is not a predefined value.
- String
- Float
- Int
- Values
using UltimateAttributesPack;
public class PredefinedValuesExample : MonoBehaviour
{
// PredefinedValues attribute displays a button at the right of the variable to open a popup menu and select one of multiples predefined values setted in params
// The value of the variable can be changed to a value that is not predefined in the params
[PredefinedValues(0, 2.5f, 5f, 10f, 33.3f)]
public float predefinedFloatValue;
// It also works with int and string
[PredefinedValues(0, 2, 5)]
public int predefinedIntValue;
[PredefinedValues(0, "Example Text", "Second Example Text")]
public string predefinedStringValue;
}
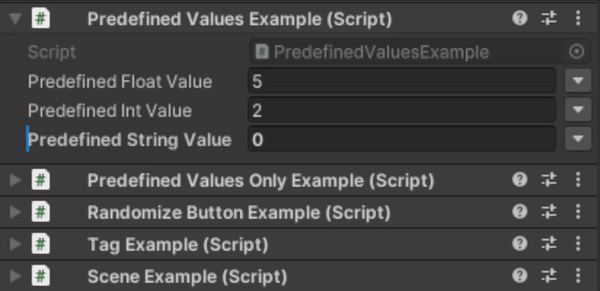
PredefinedValuesOnly
Displays a popup in the inspector to select a predefined value in parameters. The variable can't be changed to a value that is not a predefined value.
- String
- Float
- Int
- Values
using UltimateAttributesPack;
public class PredefinedValuesOnlyExample : MonoBehaviour
{
// PredefinedValuesOnly attribute displays a popup menu to select one of multiples predefined values setted in params
// The value of the variable can't be changed to a value that is not predefined in the params
[PredefinedValuesOnly(0, 2.5f, 5f, 10f, 33.3f)]
public float predefinedFloatValue;
// It also works with int and string
[PredefinedValuesOnly(0, 2, 5)]
public int predefinedIntValue;
[PredefinedValuesOnly(0, "Example Text", "Second Example Text")]
public string predefinedStringValue;
}
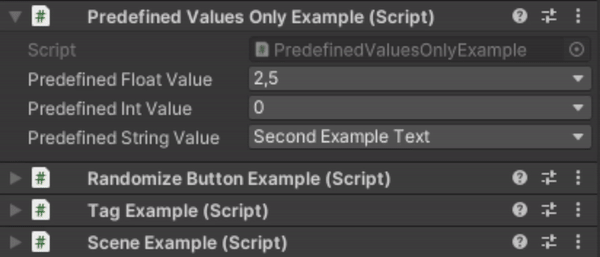